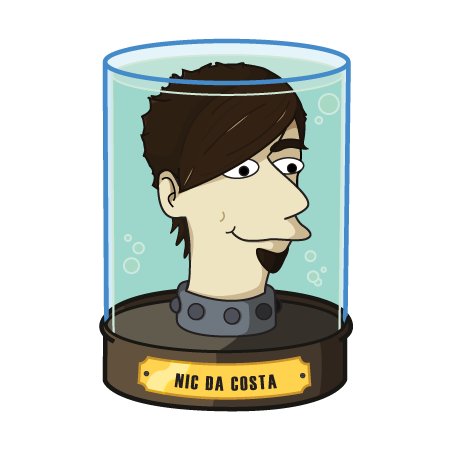
All platforms have their quirks and hacks...here are some and their solutions to help get you started
A core feature for all responsive mobile sites
@media all and (orientation: landscape) { /* only apply styles for landscape */ }
"The ‘orientation’ media feature is ‘portrait’ when the value of the ‘height’ media feature is greater than or equal to the value of the ‘width’ media feature. Otherwise ‘orientation’ is ‘landscape’".
Android Keyboard...
The keyboard is part of the screen real estate.
Fetching window.innerHeight
will be different when keyboard is up / down.
"The ‘orientation’ media feature is ‘portrait’ when the value of the ‘height’ media feature is greater than or equal to the value of the ‘width’ media feature. Otherwise ‘orientation’ is ‘landscape’".
Android Versions: 4.0 - 4.1.2 ( default browsers )
Aspect Ratio
"The ‘aspect-ratio’ media feature is defined as the ratio of the value of the ‘width’ media feature to the value of the ‘height’ media feature.’".
@media all and (min-aspect-ratio: 13/9) { /* only apply styles for landscape */ }
window.orientation; // 90 === landscape...?
This value is updated on orientationchange
event being fired.
Values are 0 or 180 for portrait and 90 or -90 for landscape...correct?
0 is based on the default "home" orientation. Motorolla Xoom, returns 0 as landscape.
Comparing window.innerHeight
to window.innerWidth
on orientationchange
AND onresize
Tip: Only run comparison once both events fired. Cache window.innerWeight
, only compare if width has changed. Due to Android Keyboard firing onresize event
window.document.addEventListener( 'resize' , function() { runResize = true; if ( runResize && runOrientation ) { handleChange() // compares: window.innerHeight > window.innerWidth runResize = false; runOrientation = false; } } , false );
Playing sound using HTML5 Audio on a device is easy...
if ( typeof window.Audio !== 'undefined' ) { // create new Audio Node var canHasSound = new Audio(); // set source file, then load canHasSound.src = "My_Amazing_Tunes.mp3"; canHasSound.load(); // PLAY!!!! canHasSound.play(); }
if ( typeof window.Audio !== 'undefined' ) { // create new Audio Node var canHasSound = new Audio(), isIOS = /iPhone|iPad|iPod/ig.test( window.navigator.userAgent ); //Hacks...Yay... // set source file, then load canHasSound.src = "My_Amazing_Tunes.mp3"; if ( isIOS ) { document.addEventListener( 'touchstart' , function() { canHasSound.load(); document.removeEventListener( 'touchstart' , arguments.callee , false ); } , false ); } else { canHasSound.load(); } // PLAY!!!! canHasSound.play(); }
// create new Audio Node var canHasSound = new Audio(), touchToLoad = /iPhone|iPad|iPod|/ig.test( window.navigator.userAgent ), //Hacks...Yay... touchToPlay = /iPhone|iPad|iPod|Chrome|Android x.x.x/ig.test( window.navigator.userAgent );//Moar // set source file, then load canHasSound.src = "My_Amazing_Tunes.mp3"; if ( touchToLoad ) { document.addEventListener( 'touchstart' , function() { canHasSound.load(); document.removeEventListener( 'touchstart' , arguments.callee , false ); } } , false ); } else { canHasSound.load(); } // PLAY!!!!...eventually... if ( touchToPlay ) { document.addEventListener( 'touchstart' , function(){ canHasSound.play(); document.removeEventListener( 'touchstart' , arguments.callee , false ); } , false ); } else { canHasSound.play(); }
var testSounds = { key : 'touchToLoad' , Modernizr : [ 'Audio'] , custom : function() { /* Create and Play Audio as Test */ } }; window.featurama.config.touchToLoad = { regex : [ /(iPad|iPhone|iPod)/ig , /(Chrome)/ig ] , result : true }; window.featurama.run( testSounds );
if ( window.featurama.results.touchToLoadSound ) { // .. rest of code }
The Mobile Web is constantly changing and evolving...
be future friendly and feature detect
Loads of Web API's that are available and AMAZE-BALLS!
var video = document.querySelector( 'video' ); navigator.getUserMedia( { audio: true , video : true }, function( stream ) { video.src = window.URL.createObjectURL(stream); }, onVideoFail );
window.navigator.onLine; // true
new Notification("JS In SA Talk about to begin", { iconUrl: "calendar.gif", body: "Room 101", onclose: function() { cancelReminders(event); } });
navigator.geolocation.getCurrentPosition( function( position ) { do_something( position.coords.latitude , position.coords.longitude ); });
Here is a list of many which are available today!
Full Chrome Dev Tools Features
But Only On Chrome for Android ( requires ADB )
Uses Safari Dev Tools
But Only On IOS 6 ( requires a Macbook/iMac )
Alows for a remote console on any device. Just include the required script
Just a console and objects are not expandable
Alows for a remote inspecting, live reload, screenshots.
On Android and IOS ( requires instalation of app on device )
npm install weinre -g
All that is missing is a JavaScript console and being able to remote debug with breakpoints...